Interrupt Service Routine (ISR)
UART layer
UART-Write - The PIC18F4550 microcontroller places a character on the data bus for the UART to read.
Asynchronous Communication
Registers of EUSART
1. TXSTA (Transmit Status and Control Register)

2. RCSTA (Receive Status and Control Register)

3. TXREG (EUSART Transmit Register)
4. RCREG (EUSART Receive Register)
5. PIR1 (Peripheral Interrupt Request Register 1)

6. PIE1 (Peripheral Interrupt Enable Register 1)

7. BAUDCON (Baud Rate Control Register)

8. SPBRG & SPBRGH (EUSART Baud Rate Generator Register Low Byte and High Byte)
Baud Rate:
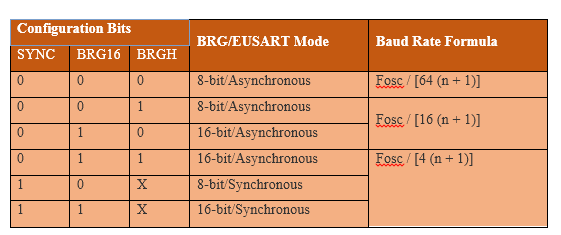
Here :

Applications
/* Name : main.c * Purpose : Source code for UART Interfacing with PIC18F4550. * Author : Gemicates * Date : 2017-06-23 * Website : www.gemicates.org * Revision : None */ #include<xc.h> // Header file for PIC18F4550 #define _XTAL_FREQ 12000000 // 12MHz #pragma config FOSC = HS // Oscillator Selection bits (HS oscillator (HS)) #pragma config WDT = OFF // HW Disabled - SW Controlled #pragma config WDT = OFF // HW Enabled - SW Disabled #pragma config BOR = OFF // Brown out reset disabled<br><br>#pragma config BOR = SOFT // Brown out reset controlled by SBOREN<br><br>#pragma config BOR = ON_ACTIVE // Enabled when the device is not in SLEEP, SBOREN bit is disabled<br><br>#pragma config BOR = ON // Brown out reset enabled, SBOREN bit is disabled #pragma config LVP = OFF // Low voltage programming mode OFF #pragma config LVP = OFF // Low voltage programming mode ON #pragma config CPD = ON // Data EEPROM Code protection ON #pragma config WRTD = OFF // Data EEPROM Write protection OFF void main() { TRISC7 = 1; // RC7(RX) configured as i/p TRISC6 = 1; // RC6(TX) configured as o/p TXSTA = 0X20; // Transmission Enable(TXEN=1,SYNC=0,BRGH=1) RCSTA = 0X90; // Rception Enable (SPEN=1,CREN=1) BAUDCON = 0X40; // Baudrate control Register SPBRG = 0X12; // 9600 brgh=0 TXREG = 'E'; // Load the character to be transmitted while(TRMT); // Wait here till transmission is complete while(1) { if(RCIF==1) // Set when a character is received { RCIF=0; // Clear Receive interrupt flag bit RCREG = TXREG ; // Retransmit the received character while(TRMT); // Wait here till transmission is complete } } }